I have a code that takes a form's email field, looks up the associated contact on the wix website and then autofill other fields on the same form.
I have a temporary collection called AllContacts (see image).
When I test the code and type "tim11@server.com" it returns "no contact" It is definitely there.
the page code I have follows:
import wixCRM from 'wix-crm';
import wixData from 'wix-data';
//import wixForms from 'wix-forms';
$w.onReady(function () {
const emailField = $w('#emailInput');
const firstNameField = $w('#firstName');
const lastNameField = $w('#lastName');
emailField.onBlur(() => {
const email = emailField.value;
if (email) {
lookupContactByEmail(email)
.then(contact => {
if (contact) {
firstNameField.value = contact.firstName;
lastNameField.value = contact.lastName;
} else {
console.log('No contact found with that email.');
}
})
.catch(error => {
console.error('Error looking up contact:', error);
});
}
});
});
function lookupContactByEmail(email) {
return wixData.query('AllContacts')
.eq('primaryInfo.email', email)
.find()
.then(results => {
if (results.items.length > 0) {
return results.items[0];
} else {
return null;
}
})
.catch(error => {
console.error('Error querying Contacts:', error);
throw error;
});
}
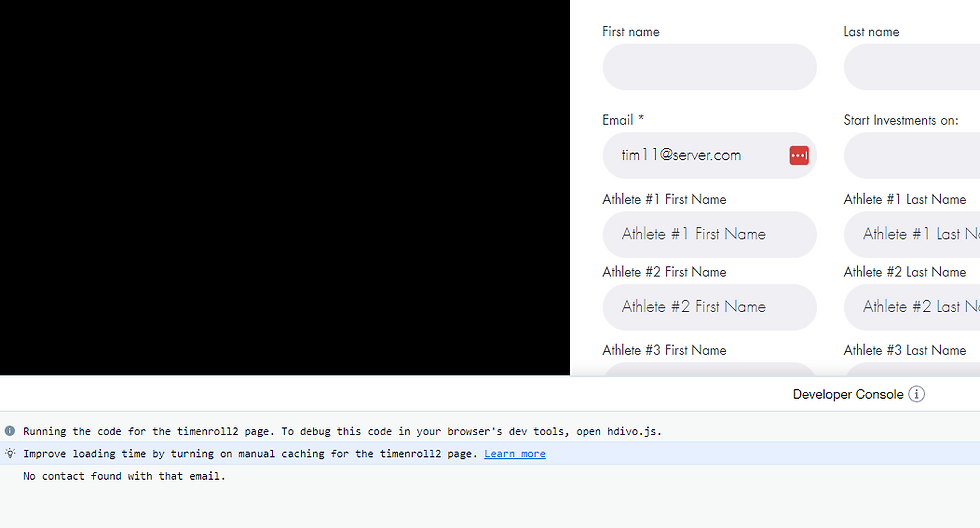
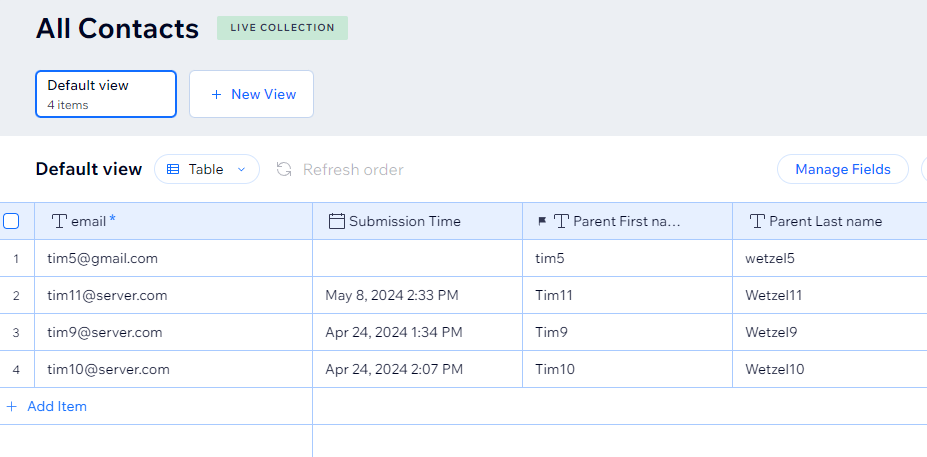
Thanks for the help!
Hi Tim, Since generally your code looks okay, I would first check this line as the cause of the empty query result:
.eq('primaryInfo.email', email)
Either:
There is an issue with the field name, maybe it is just 'email'?
There isn't an exact match between email and the value of the field (maybe due to spacing, capitalization etc. You can try using .contains instead of .eq to avoid this.
If it isn't one of those two I would need to go back to the drawing board. Best, Eitan